# | include: false
import numpy as np
import pandas as pd
256852) np.random.seed(
Files, Folders & OS (Need) Exercises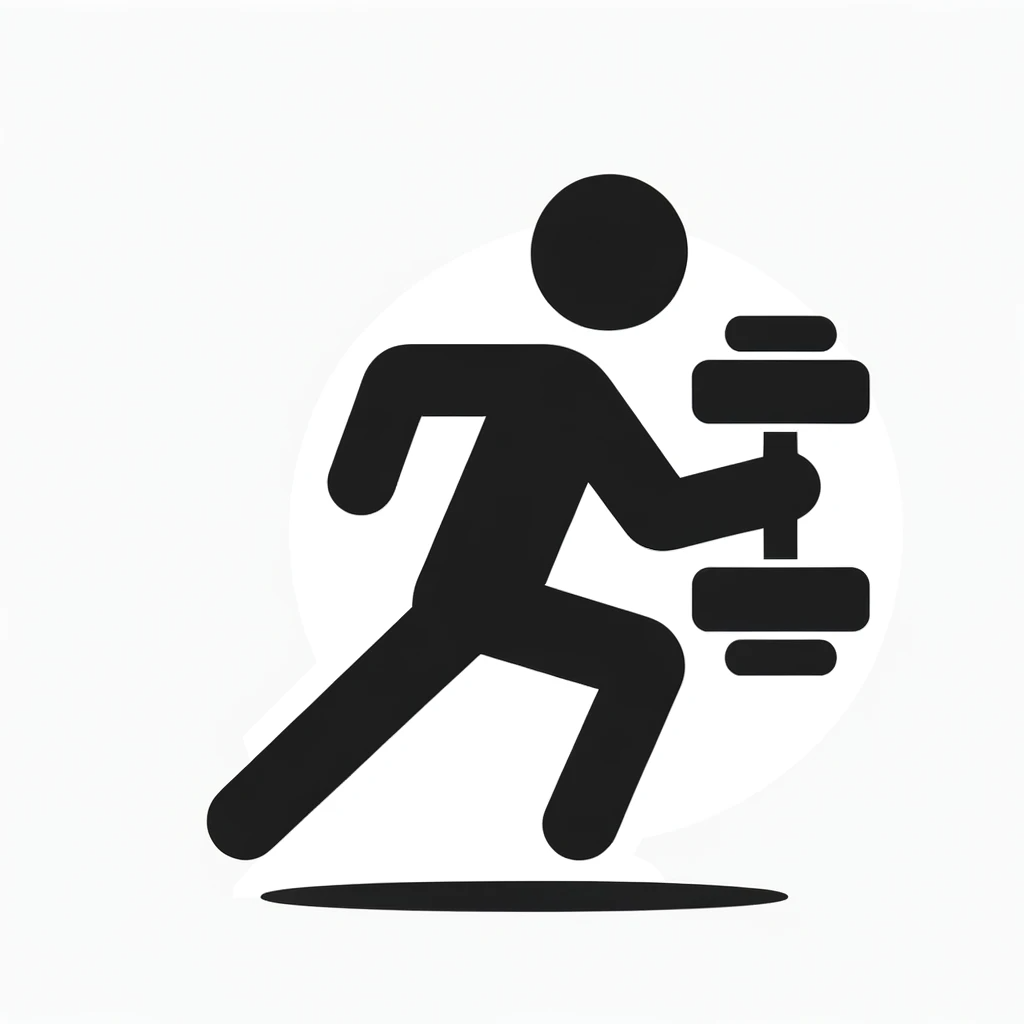
Exercise 1 (Tidying a collaboration) ☻
You are a member of an international team analysing environmental pollution. The project involves ten cities: Cairo, Dhaka, Jakarta, Karachi, Manila, Melbourne, Osaka, Shanghai, Singapore, and Tokyo.
Your task is to process and organise data from various environmental measurements. The provided zip file, os-collaboration-exercise-data.zip, contains all relevant data files for the past year.
Things to note
- Data Files: Each data file is named in the format
month-date_city.txt
(e.g.,may-10_singapore.txt
). - Data Collection: Data was recorded sporadically; not all days of each month have corresponding data files. The dates of data collection vary across the cities.
- Exclusion of Files: Ignore any non-text files, such as those in
.pdf
,.png
, or.jpg
formats.
We like to convert the filename to a more useful numerical format. Specifically, we want to convert
month-date-city.txt
. (i.e.may-10-singapore.txt
) to amm-dd-city.txt
(i.e.05-10-singapore.txt
) format.Using the following dictionary (or otherwise), write a snippet of Python code to convert
'oct-08_singapore.txt'
to10-08-singapore.txt
.{'jan': '01', 'feb': '02', 'mar': '03', 'apr': '04', 'may': '05', 'jun': '06', 'jul': '07', 'aug': '08', 'sep': '09', 'oct': '10', 'nov': '11', 'dec': '12' }
- Incorporate your previous code into a function named
rename_my_file(old_file_name)
that accepts the old filename as the argument and returns the new file name.
Use a
for
loop to apply the functionrename_my_file()
to the file list below.'oct-08_singapore.txt', 'jul-10_cairo.txt', 'may-15_dhaka.txt', ['may-13_cairo.txt', 'oct-21_cairo.txt', 'jan-10_singapore.txt', 'jun-20_tokyo.txt', 'aug-06_jakarta.txt', 'dec-21_karachi.txt', 'jan-01_tokyo.txt']
Print out your progress in the form
old-file-name ----> new-file-name
With the help of
glob
, userename_my_file()
to rename all the.txt
files in the folder.Note that you might have to adjust your function
rename_my_file()
to accommodate the already renamed files.
Use a
for
loop to create a folder for each city.
The list of cities is provided below for your convenience.'Cairo', 'Dhaka', 'Jakarta', 'Karachi', 'Manila', ['Melbourne', 'Osaka', 'Shanghai', 'Singapore', 'Tokyo']
- Use
glob
to list all the files from Cairo. - Now use a
for
loop andshutil.copy()
to copy all the files related to Cairo to the corresponding folder you created.
- Tweak your code to move all the files to the corresponding folders of all the cities.