# | include: false
import numpy as np
import pandas as pd
256852) np.random.seed(
Functions (Good) Exercises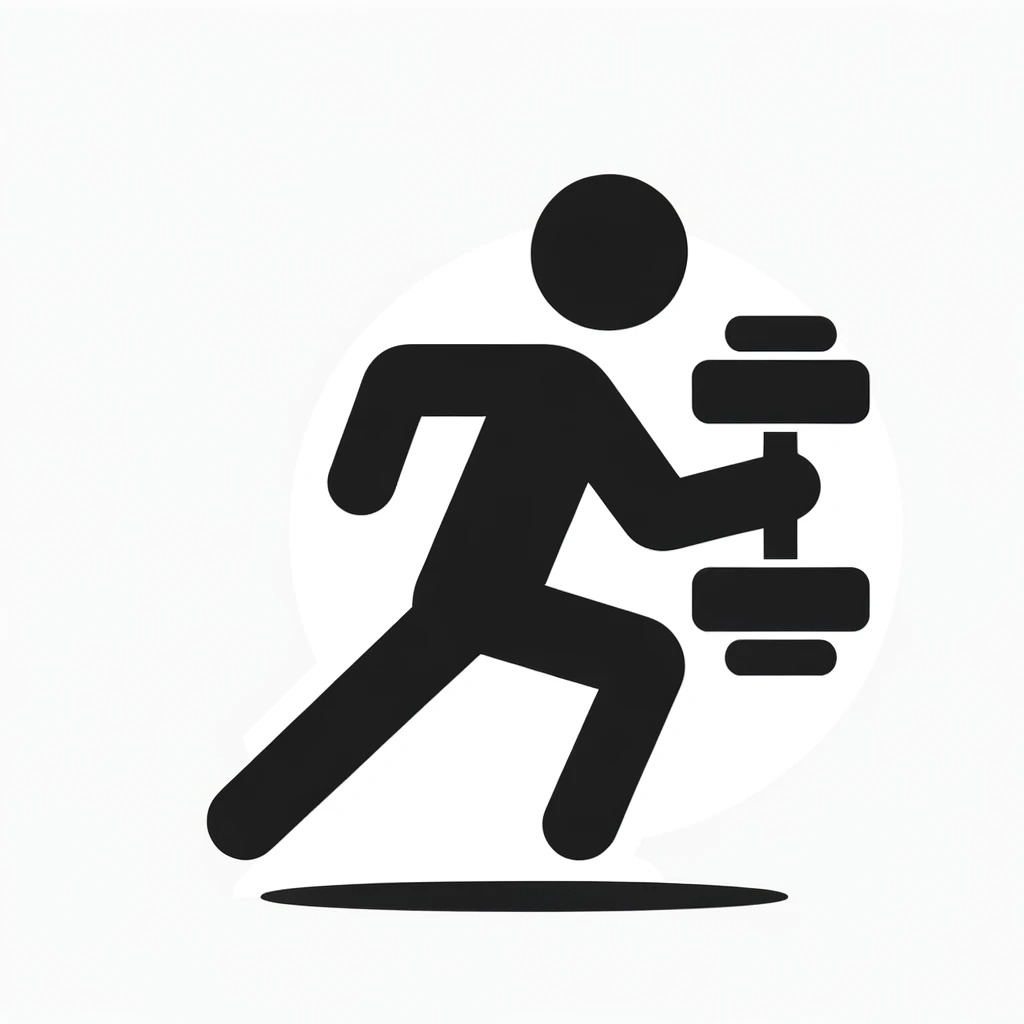
Exercise 1 (Celsius to Fahrenheit or Kelvin) ☻
- Develop a function named
convert_celsius()
to convert temperatures from Celsius to either Fahrenheit or Kelvin. - The function should take two arguments:
temperature_celsius
: The temperature in Celsius.target_scale
(string): The target scale for conversion, with the default value set to'Fahrenheit'
.
- The function should return the temperature in Kelvin if
target_scale
is'Kelvin'
; otherwise, it should return the temperature in Fahrenheit.
Exercise 2 (Fahrenheit to Celsius or Kelvin) ☻
- Develop a function called
convert_fahrenheit()
for converting temperatures from Fahrenheit to either Celsius or Kelvin. - The function should take two arguments:
temperature_fahrenheit
: The temperature in Fahrenheit.target_scale
(string): The target scale for conversion, defaulting to'Celsius'
.
- The function should return the temperature in Kelvin if
target_scale
is'Kelvin'
; otherwise, return it in Celsius.
Exercise 3 (General Temperature Conversion) ☻
- Implement a function named
convert_temperature()
to perform general temperature conversions. - The function should take three arguments:
temperature
: The temperature to be converted.source_scale
(string): The scale of the input temperature (either'Celsius'
,'Fahrenheit'
, or'Kelvin'
).target_scale
(string): The desired scale for the output temperature.
- Remember to reuse your previous functions!