# | include: false
import numpy as np
import pandas as pd
256852) np.random.seed(
Storing Data (Need) Exercises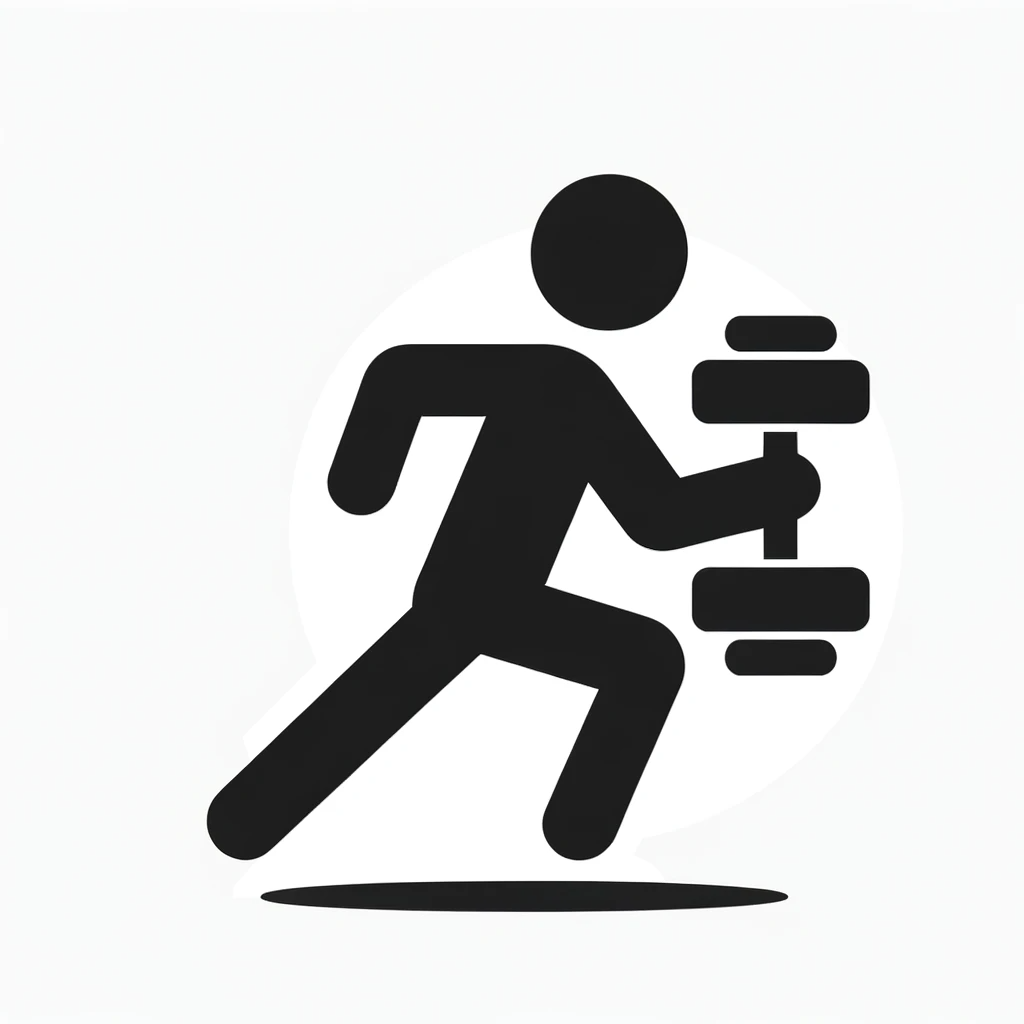
Exercise 1 (Total recall?) ☻
Purely from memory, jot down:
- Two similarities between lists and arrays.
- Two differences between lists and arrays.
- What is a dictionary?
If you cannot recall the answers, please refer to the course notes and put this information you could not recall in italics.
Exercise 2 (Indexing) ☻
Modify the following code to print out all elements with an odd number. I have done the one corresponding to i9
for you.
= ["a1", "b2", "c3", "d4", "e5", "f6", "g7", "h8", "i9", "j10"]
py_list # Prints 'a1'
# Prints 'c3'
# Prints 'e5'
# Prints 'g7'
print(py_list[8]) # Prints 'i9'
i9
Exercise 3 (Index again) ☻
Given the following list in Python:
= ['Hydrogen',
elements 'Helium', 'Lithium',
'Beryllium', 'Boron', 'Carbon',
'Nitrogen', 'Oxygen',
'Fluorine',
'Neon']
- Access and print the element at index 4 using forward indexing.
- Access and print the element at index 4 from the end of the list using reverse indexing.
Exercise 4 (How many ones) ☻
Use the concepts you learned in this chapter to determine the number of 1’s in the following list of numbers.
=[45, 60, 1, 30, 96, 1, 96, 57, 16, 1,
numbers99, 62, 86, 43, 42, 60, 59, 1, 1, 35,
83, 47, 34, 28, 68, 23, 22, 92, 1, 79,
1, 29, 94, 72, 46, 47, 1, 74, 32, 20,
8, 37, 35, 1, 89, 29, 86, 19, 43, 61]
Here are some hints:
- Use a NumPy array.
- Ask a question.
False
is considered0
, andTrue
is considered1
bysum()
.