# | include: false
import numpy as np
import pandas as pd
256852) np.random.seed(
Loops (Need)
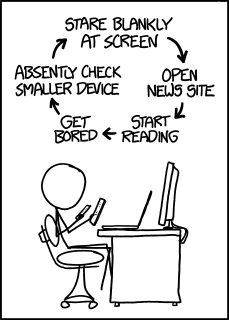
What to expect in this chapter
One of the most powerful features of programming is the ability to repeat a task over and over again. This can be as mundane as renaming a set of files or more involved, like running the same statistical analysis on many experimental data sets. While humans quickly become tired (and bored) with such tasks, computers excel at mindless repetitive tasks. This section will show you how to repeat things in Python. This topic is called loops or iterations and is one of the most valuable features of any programming language.
Python offers two mechanisms for looping. One is the for
statement, and the other is the while
statement. We will spend more time on the former as it is the more used of the two.
1 The for
iterator
Let me quickly show you a few examples of using a for
loop.
Let’s say I want to print a message corresponding to every superhero in the following list.
= ["Natasha Romanoff", "Tony Stark", "Stephen Strange"] real_names
Here is one (poor) way of doing this.
=real_names[0]
nameprint(f"{name} is a Marvel superhero!")
=real_names[1]
nameprint(f"{name} is a Marvel superhero!")
=real_names[2]
nameprint(f"{name} is a Marvel superhero!")
Natasha Romanoff is a Marvel superhero!
Tony Stark is a Marvel superhero!
Stephen Strange is a Marvel superhero!
This way of programming is not very good because:
- it does not scale very well (imagine if you have a 100 names!),
- it is cumbersome to make changes, you have to do it three times.
- it is highly error prone, since you need to type something new (even if you are copying and pasting most of it).
This is where a for
loop can come in handy.
1.1 for
with a list
I can achieve the same result using a for
loop as follows:
for name in real_names:
print(f"{name} is a Marvel superhero!")
Natasha Romanoff is a Marvel superhero!
Tony Stark is a Marvel superhero!
Stephen Strange is a Marvel superhero!
Notice the structure of the for
loop;
- it goes through the list and assigns
name
the value of each element of the list. - it then runs the code-block using this value of
name
. - the code block is deginted by using
:
and tabs like withif
.
Remember that for
can be used to directly loop through a list.
By the way, there is nothing special about the names of the variables I have used. The following will work too. However, it will not be very readable because x
is a bit cryptic in this context.
for x in real_names:
print(f"{x} is a Marvel superhero!")
1.2 for
with enumerate
Let’s say we want to do a bit more, like using the information in both of the following lists.
= ["Black Widow", "Iron Man", "Doctor Strange"]
super_names = ["Natasha Romanoff", "Tony Stark", "Stephen Strange"] real_names
Since the for
loop only accepts one list, we need to do something else to access the data in both lists. One option is to use enumerate()
. Let me first show you how enumerate()
works.
for count, name in enumerate(real_names):
print(f'{count}: {name} is a Marvel superhero!')
0: Natasha Romanoff is a Marvel superhero!
1: Tony Stark is a Marvel superhero!
2: Stephen Strange is a Marvel superhero!
You can think of enumerate()
as something that keeps count. In the above example, enumerate()
not only gives the elements of the list, it also gives you a number (that is stored in count
).
Other than counting, we can use the count given by enumerate()
to index the other list!
for index, name in enumerate(real_names):
= super_names[index]
superhero_name print(f'{name} is {superhero_name}!')
Natasha Romanoff is Black Widow!
Tony Stark is Iron Man!
Stephen Strange is Doctor Strange!
Before we move on, I would like to highlight two things:
Notice how I changed the variable name used with
enumerate()
to (count
andindex
) to match their logical use. This makes it easy to iimediately see what you are doing (i.e., the intention) wiht the code. Python does not really care abot this, but remember that we write programmes for humans!Although by default,
enumerate()
starts counting from 0, we can easily change it to start at another value, say 100.for count, name in enumerate(real_names, 100): print(f'{count}: {name} is a Marvel superhero!')
100: Natasha Romanoff is a Marvel superhero! 101: Tony Stark is a Marvel superhero! 102: Stephen Strange is a Marvel superhero!
Remember that for
can be combined with enumerate()
to count while looping through a list.
1.3 for
with range
Yet, another way to achieve the result above is by using the function range()
. But, first lets see what range()
can do.
-
for i in range(5): print(i)
We can use
range()
to get thefor
loop to run a given number of loops (5 in this example).0 1 2 3 4
-
for i in range(5, 10): print(i)
We can tailor the starting and ending values…
5 6 7 8 9
-
for i in range(1, 10, 3): print(i)
We can even adjust the step size.
1 4 7
Note:
- Functions like
range()
andenumerate()
only work with looping structures. range()
always ends one short of the ending number.
Now, let’s return to our initial problem of printing superhero names. We can use range()
as follows:
for i in range(len(real_names)):
= real_names[i]
real_name = super_names[i]
super_name print(f"{real_name} is Marvel's {super_name}!")
Natasha Romanoff is Marvel's Black Widow!
Tony Stark is Marvel's Iron Man!
Stephen Strange is Marvel's Doctor Strange!
Notice that I have used the len(real_names)
to get how many times the loop should run.
Remember that for
can be run a given number of times using range()
.
2 while
= 0
number
while number < 5:
print(number)
+= 1 number
Now let’s take a quick look at the while
loop. A while
loop is set up so that it keeps on running while a condition is True
. So the while
loop checks the condition at the start and begins another loop if it is True
.
In the adjoining example the loop keeps on going while the number is less than 5.
A useful feature of the while
loop is that you do not need to know beforehand how many iterations are needed.
0
1
2
3
4